Open Shading Language
Cycles Only
It is also possible to create your own nodes using Open Shading Language (OSL). These nodes will only work with the CPU and OptiX rendering backend.
Для включения этой возможности отметьте галочку Open Shading Language в панели Визуализация.
Примечание
Some OSL features are not available when using the OptiX backend. Examples include:
Memory usage reductions offered by features like on-demand texture loading and mip-mapping are not available.
Texture lookups require OSL to be able to determine a constant image file path for each texture call.
Some noise functions are not available. Examples include Cell, Simplex, and Gabor.
The trace function is not functional. As a result of this, the Ambient Occlusion and Bevel nodes do not work.
Узел «Скрипт»
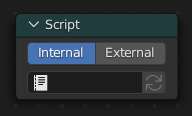
OSL was designed for node-based shading, and each OSL shader corresponds to one node in a node setup. To add an OSL shader, add a script node and link it to a text data-block or an external file. Input and output sockets will be created from the shader parameters on clicking the update button in the Node or the Text editor.
Шейдеры OSL могут быть связаны с узлом несколькими различными способами. При использовании режима Встроенный для хранения шейдера OSL будет использоваться текстовый блок данных, а байт-код OSO будет сохранён в самом узле. Это полезно для распространения blend-файла без зависимостей.
Режим Внешний может использоваться для указания файла .osl
на диске, который будет автоматически скомпилирован в файл .oso
и помещён в тот же каталог. Так же возможно определить путь непосредственно к файлу .oso
, который в таком случае будет использоваться напрямую и должен будет компилироваться пользователем самостоятельно. Третьим вариантом можно указать просто имя модуля, который будет искаться по пути поиска шейдеров.
Шейдеры ищутся в том же самом месте, где расположены все остальные скрипты или другая конфигурация:
- Linux
$HOME/.config/blender/4.0/shaders/
- Windows
C:\Users\$user\AppData\Roaming\Blender Foundation\Blender\4.0\shaders\
- macOS
/Users/$USER/Library/Application Support/Blender/4.0/shaders/
Совет
Для использования в реальной работе, мы предлагаем использовать узел группы, обёртывающий узлы скрипта и ссылаться на него из других blend-файлов. Это упростит внесение изменений в узел после добавления или удаления сокетов, без необходимости в обновлении узлов скриптов во всех файлах.
Написание шейдеров
For more details on how to write shaders, see the OSL specification. Here is a simple example:
shader simple_material(
color Diffuse_Color = color(0.6, 0.8, 0.6),
float Noise_Factor = 0.5,
output closure color BSDF = diffuse(N))
{
color material_color = Diffuse_Color * mix(1.0, noise(P * 10.0), Noise_Factor);
BSDF = material_color * diffuse(N);
}
Замыкания
OSL is different from, for example, RSL or GLSL, in that it does not have a light loop. There is no access to lights in the scene, and the material must be built from closures that are implemented in the renderer itself. This is more limited, but also makes it possible for the renderer to do optimizations and ensure all shaders can be importance sampled.
The available closures in Cycles correspond to the shader nodes and their sockets; for more details on what they do and the meaning of the parameters, see the shader nodes manual.
BSDF
diffuse(N)
oren_nayar(N, roughness)
diffuse_ramp(N, colors[8])
phong_ramp(N, exponent, colors[8])
diffuse_toon(N, size, smooth)
glossy_toon(N, size, smooth)
translucent(N)
reflection(N)
refraction(N, ior)
transparent()
microfacet_ggx(N, roughness)
microfacet_ggx_aniso(N, T, ax, ay)
microfacet_ggx_refraction(N, roughness, ior)
microfacet_beckmann(N, roughness)
microfacet_beckmann_aniso(N, T, ax, ay)
microfacet_beckmann_refraction(N, roughness, ior)
ashikhmin_shirley(N, T, ax, ay)
ashikhmin_velvet(N, roughness)
Волосы
hair_reflection(N, roughnessu, roughnessv, T, offset)
hair_transmission(N, roughnessu, roughnessv, T, offset)
principled_hair(N, absorption, roughness, radial_roughness, coat, offset, IOR)
BSSRDF
bssrdf_cubic(N, radius, texture_blur, sharpness)
bssrdf_gaussian(N, radius, texture_blur)
Volume (объём)
henyey_greenstein(g)
absorption()
Other
emission()
ambient_occlusion()
holdout()
background()
Атрибуты
Geometry attributes can be read through the getattribute()
function.
This includes UV maps, color attributes and any attributes output from geometry nodes.
The following built-in attributes are available through getattribute()
as well.
geom:generated
Automatically generated texture coordinates, from undeformed mesh.
geom:uv
UV-развёртка по умолчанию.
geom:tangent
Default tangent vector along surface, in object space.
geom:undisplaced
Position before displacement, in object space.
geom:dupli_generated
For instances, generated coordinate from instancer object.
geom:dupli_uv
For instances, UV coordinate from instancer object.
geom:trianglevertices
Three vertex coordinates of the triangle.
geom:numpolyvertices
Количество вершим в многоугольнике (на текущий момент всегда возвращает три вершины).
geom:polyvertices
Массив с координатами вершин многоугольника (на текущий момент в массиве всегда три вершины).
geom:name
Имя объекта.
geom:is_smooth
Is mesh face smooth or flat shaded.
geom:is_curve
Is object a curve or not.
geom:curve_intercept
0..1 coordinate for point along the curve, from root to tip.
geom:curve_thickness
Thickness of the curve in object space.
geom:curve_length
Length of the curve in object space.
geom:curve_tangent_normal
Касательная нормали пряди.
geom:is_point
Is point in a point cloud or not.
geom:point_radius
Radius of point in point cloud.
geom:point_position
Center position of point in point cloud.
geom:point_random
Random number, different for every point in point cloud.
path:ray_length
Расстояние, пройденное лучом с момента последнего попадания в препятствие.
object:random
Random number, different for every object instance.
object:index
Object unique instance index.
object:location
Местоположение объекта.
material:index
Material unique index number.
particle:index
Particle unique instance number.
particle:age
Возраст частицы в кадрах.
particle:lifetime
Общее время жизни частицы в кадрах.
particle:location
Местоположение частицы.
particle:size
Размер частицы.
particle:velocity
Скорость частицы.
particle:angular_velocity
Угловая скорость частицы.
Трассировка
CPU Only
Мы поддерживаем функцию trace(point pos, vector dir, ...)
для трассировки лучей из шейдеров OSL. Параметр «shade» на текущий момент не поддерживается, но атрибуты объекта, с которым произошло столкновение луча, могут быть получены через функцию getmessage("trace", ..)
. Подробности по использованию этого механизма смотрите в спецификации OSL.
Эта функция не может использоваться вместо освещения; основная её цель – позволить шейдерам «щупать» ближайшую геометрию, например, чтобы применить проецируемую текстуру, которая может быть заблокирована геометрией, сделать открытую геометрию более «изношенной» или применить ещё какие-нибудь эффекты, подобные ambient occlusion.
Metadata (метаданные)
Metadata on parameters controls their display in the user interface. The following metadata is supported:
[[ string label = "My Label" ]]
Name of parameter in in the user interface
[[ string widget = "null" ]]
Hide parameter in the user interface.
[[ string widget = "boolean" ]]
and[[ string widget = "checkbox" ]]
Display integer parameter as a boolean checkbox.